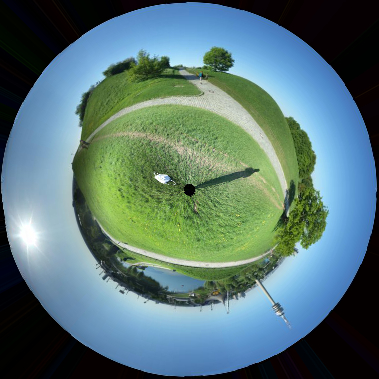
Today I saw (again) Filipe Calegario's nice photos and instructions for making polar panoramas. To show how to warp images with Python I wrote a Python version of the polar warp. Here's a complete code example that uses scipy.ndimage (except for the final hole filling/interpolation).
import Image
from numpy import *
from scipy.ndimage import geometric_transform
from scipy.misc import imsave
def polar_warp(im,sz,r):
""" Warp an image to a square polar version. """
h,w = im.shape[:2]
def polar_2_rect(xy):
""" Convert polar coordinates to coordinates in
the original image for geometric_transform. """
x,y = float(xy[1]),float(xy[0])
theta = 0.5*arctan2(x-sz/2,y-sz/2)
R = sqrt( (x-sz/2)*(x-sz/2) + (y-sz/2)*(y-sz/2) ) - r
return (2*h*R/(sz-2*r),w/2+theta*w/pi+pi/2)
# if color image, warp each channel, otherwise there's just one
if len(im.shape)==3:
warped = zeros((sz,sz,3))
for i in range(3):
warped[:,:,i] = geometric_transform(im[:,:,i],polar_2_rect,output_shape=(sz,sz),mode='nearest')
else:
warped = geometric_transform(im,polar_2_rect,output_shape=(sz,sz),mode='nearest')
return warped
if __name__ == "__main__":
# load image from http://www.filipecalegario.com/comppho/3assignment/
im = array(Image.open('autostitch-stitch.jpg'))
h,w = im.shape[:2]
# flip vertical to make math easier for warp
im = flipud(im)
# warp image
wim = uint8(polar_warp(im,h,5))
# save
imsave('polar.png',wim)
This reads a regular 360 panorama (in this example the "autostitch-stitch.jpg" file), flips the image and warps so that the sky faces out. Save this as a file and run from the command line and you should get an image like the one above.
Here's the file if you don't want to copy/paste the above.