Arnold's cat map is a fun mapping to randomize images by shuffling the pixels around. It distorts the image by shearing and then moves the pieces outside the original image region back using the integer mod function. Applied iteratively, this results in randomizing the image in a way that eventually returns the original. Here's a code example that iteratively applies the mapping and saves intermediate images: ![]()
![]()
![]()
![]()
![]()
Cat image (128*128) at iteration 0, 1, 64, 126, 127, 128. (original image here, credit CC Flickr @outlier*)
Tradition has it that this mapping should be applied to pictures of cats. (The name comes from Vladimir Arnold, who demonstrated the mapping on an image of a cat)
import Image
from numpy import *
from scipy.misc import lena,imsave
# load image
im = array(Image.open("cat.jpg"))
N = im.shape[0]
# create x and y components of Arnold's cat mapping
x,y = meshgrid(range(N),range(N))
xmap = (2*x+y) % N
ymap = (x+y) % N
for i in xrange(N+1):
imsave("cat_{0}.png".format(i),im)
im = im[xmap,ymap]
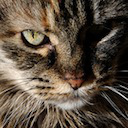
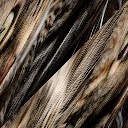
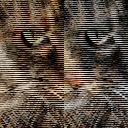
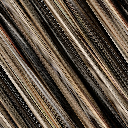
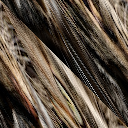
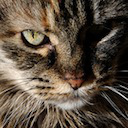